gdb测试代码
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58
| #include <iostream> using namespace std; #include <string> #include <mutex> #include <thread>
class test { public: test() { } ~test() { } static void do_work1() { cout << "do work 1" << endl; std::this_thread::sleep_for(std::chrono::seconds(2)); cout << "do work 1 exit" << endl; } void do_work2() { cout << "do work 2" << endl; std::this_thread::sleep_for(std::chrono::seconds(2)); cout << "do work 2 exit" << endl; } void do_work3(string arg, int x, int y) { cout << "do work 3 x=" << x << "y=" << y << endl; std::this_thread::sleep_for(std::chrono::seconds(2)); cout << "do work 3 exit" << endl; } };
void test_thread(int &data) { cout << "thread data=" << data << endl; std::this_thread::sleep_for(std::chrono::seconds(2)); cout << "do datathread exit" << endl;
} int main() { int data=10; thread t1(&test_thread,ref(data)); thread t2(&test::do_work1); test t; thread t3(&test::do_work2,t); thread t4(&test::do_work3,t,"string",10,20); t1.join(); t2.join(); t3.join(); t4.join();
return 0; }
|
线程相关命令
info threads |
查看所有线程信息 |
thread find |
查找线程 |
thread num |
切换线程 |
b breakpoint thread id |
为线程设置端点 |
thread apply |
为线程执行命令 |
set scheduler-locking off/on/step |
锁定线程 |
info threads
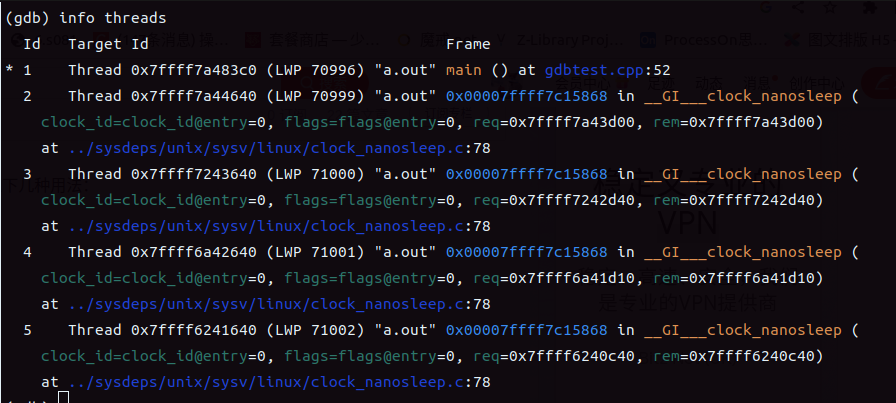
thread 0x7ff..(线程地址) LWP(线程pid,light weight process) at (在某一行) in (在哪个函数停下来)
thread num
切换到2号线程
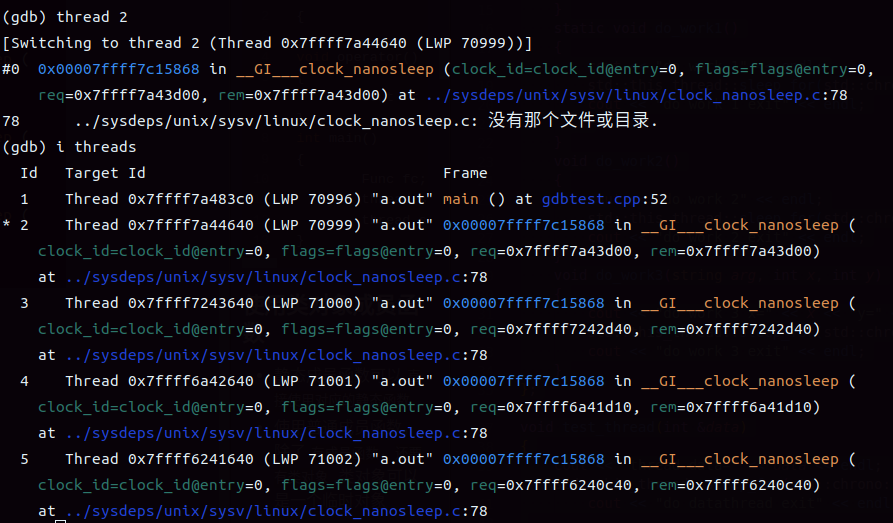
thread find
使用 线程地址
,lwp
,线程名字
都可以来查找线程
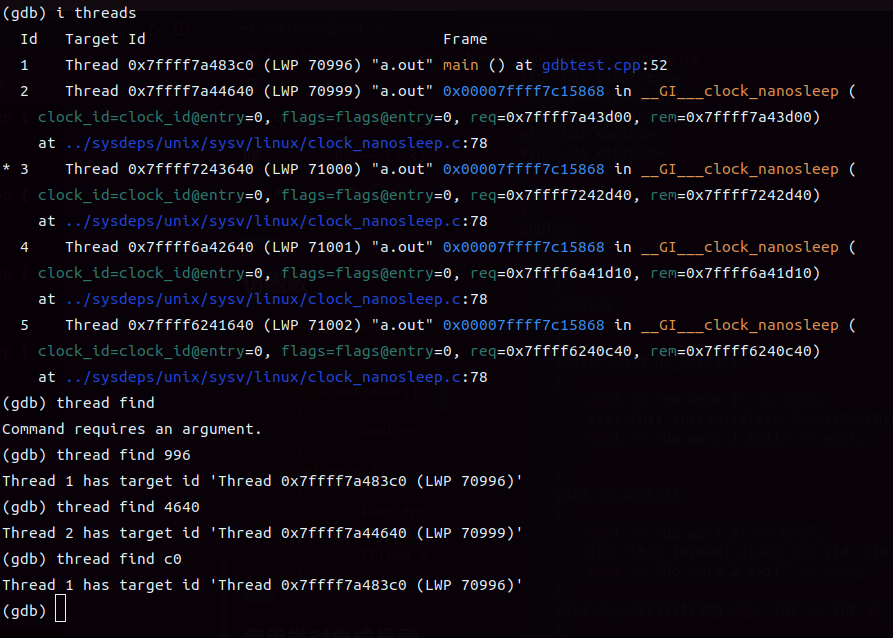
thread name
给线程改名
thread name 2
为线程设置断点
b 29 thread 5
thread apply
thread apply num (执行普通gdb的命令)
这样就不用切换线程了
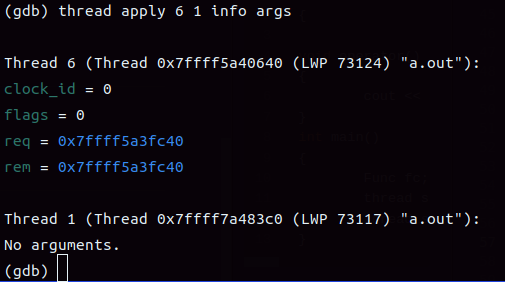
查看所有线程的调用栈(线程死锁了,就可以查看不同线程的函数调用情况来解决)
1 2 3 4 5 6 7 8
| thread apply 1-5 bt
thread apply all i args
thread apply -s all i args
|